Determining the Existence of a Directory Entry
The following static methods of the Files class test the existence or nonexistence of a directory entry denoted by a path.
static boolean exists(Path path, LinkOption… options)
static boolean notExists(Path path, LinkOption… options)
The first method tests whether a directory entry exists. The second method tests whether the directory entry denoted by this path does not exist.
These methods normalize the path, and do not follow symbolic links if the enum constant LinkOption.NOFOLLOW_LINKS is specified for the options variable arity parameter.
These methods are not complements of each other. Both return false if they are not able to determine whether a directory entry exists or not. This can occur due to lack of access permissions.
Note that the result returned by these methods is immediately outdated. The outcome of subsequent access of the directory entry is unpredictable, as concurrently running threads might change the conditions after the method returns.
Although a Path object can represent a hypothetical path, ultimately the existence of the directory entry it denotes must be verified by interacting with the file system.
Given the following directory hierarchy, the code below demonstrates what result is returned by the exists() and notExists() methods of the Files class.
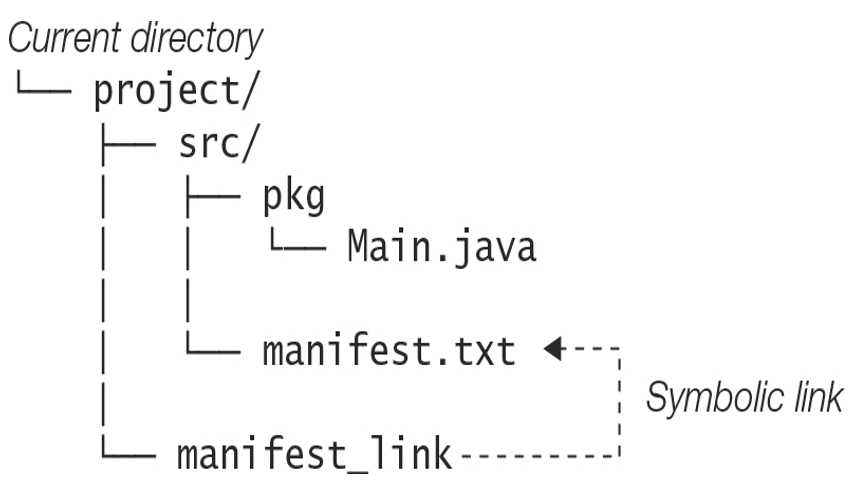
Path path1 = Path.of(“project”, “src”, “pkg”, “Main.java”);
System.out.println(Files.exists(path1)); // true
System.out.println(Files.notExists(path1)); // false
Path path2 = Path.of(“project”, “..”, “project”, “.”, “src”, “pkg”, “Main.java”);
System.out.println(Files.exists(path2)); // true
System.out.println(Files.notExists(path2)); // false
Path path3 = Path.of(“project”, “readme.txt”);
System.out.println(Files.exists(path3)); // false
System.out.println(Files.notExists(path3)); // true
Given that the path ./project/manifest_link is a symbolic link to the path ./project/ src/manifest.txt, the code below demonstrates following symbolic links in the exists() method.
Path target = Path.of(“project”, “src”, “manifest.txt”);
Path symbLink = Path.of(“project”, “manifest_link”);
boolean result4 = Files.exists(target); // (1)
boolean result5 = Files.exists(symbLink); // (2)
boolean result6 = Files.exists(symbLink, LinkOption.NOFOLLOW_LINKS); // (3)
System.out.println(“target: ” + result4); // (1a) true
System.out.println(“symbLink->target: ” + result5); // (2a) true
System.out.println(“symbLink_NOFOLLOW_LINKS: ” + result6); // (3a) true
Note that (1) and (2) above are equivalent. The existence of the target is tested at (2) as the symbolic link is followed by default. Whereas at (3), the existence of the symbolic link itself is tested, since the enum constant LinkOption.NOFOLLOW_LINKS is specified.
Leave a Reply